A* Examples
Normal A*
The A* algorithm can be run with the following.
1def normal_a_star():
2 import numpy as np
3 from gncpy.planning.a_star import AStar
4
5 # define start and end location (x, y)
6 start_pos = np.array([10, 10])
7 end_pos = np.array([50, 50])
8
9 # define grid square width/height (x, y)
10 grid_res = np.array([2, 2])
11
12 # create some obstacles
13 obstacles = np.array([])
14 w = 1
15 h = 1
16 for i in range(-10, 60):
17 if obstacles.size == 0:
18 obstacles = np.array([[i, -10, w, h]])
19 continue
20 obstacles = np.concatenate((obstacles, np.array([[i, -10, w, h]])), axis=0)
21 for i in range(-10, 60):
22 obstacles = np.concatenate((obstacles, np.array([[60, i, w, h]])), axis=0)
23 for i in range(-10, 61):
24 obstacles = np.concatenate((obstacles, np.array([[i, 60, w, h]])), axis=0)
25 for i in range(-10, 61):
26 obstacles = np.concatenate((obstacles, np.array([[-10, i, w, h]])), axis=0)
27 for i in range(-10, 40):
28 obstacles = np.concatenate((obstacles, np.array([[20, i, w, h]])), axis=0)
29 for i in range(0, 40):
30 obstacles = np.concatenate((obstacles, np.array([[40, 60 - i, w, h]])), axis=0)
31
32 # create some hazards
33 hazards = np.array([[44, 24, 8, 15, 2], [26, 36, 9, 4, 1.75]])
34
35 # define the bounds of the search region
36 min_pos = np.min(obstacles[:, 0:2], axis=0)
37 max_pos = np.max(obstacles[:, 0:2], axis=0)
38
39 # create the class, and setup the map
40 aStar = AStar()
41 aStar.set_map(min_pos, max_pos, grid_res, obstacles=obstacles, hazards=hazards)
42
43 # call the algorithm
44 path, cost, fig, frame_list = aStar.plan(
45 start_pos, end_pos, show_animation=True, save_animation=True
46 )
47
48 return frame_list
which gives this as output.
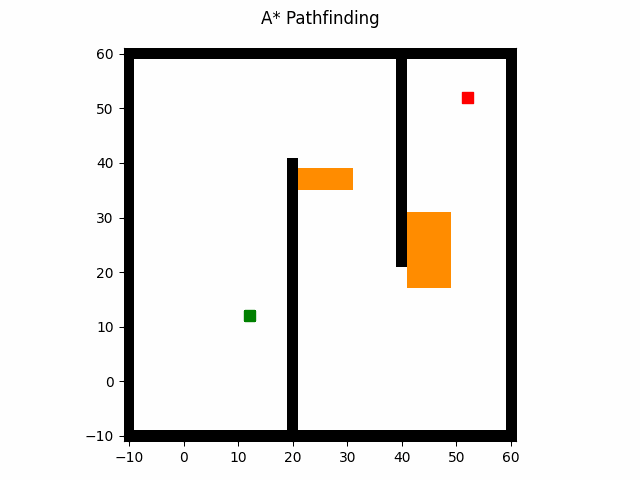
Beam Search
A modified A* algorithm called beam search can be run with the following.
1def beam_search():
2 import numpy as np
3 from gncpy.planning.a_star import AStar
4
5 # define start and end location (x, y)
6 start_pos = np.array([10, 10])
7 end_pos = np.array([50, 50])
8
9 # define grid square width/height (x, y)
10 grid_res = np.array([2, 2])
11
12 # create some obstacles
13 obstacles = np.array([])
14 w = 1
15 h = 1
16 for i in range(-10, 60):
17 if obstacles.size == 0:
18 obstacles = np.array([[i, -10, w, h]])
19 continue
20 obstacles = np.concatenate((obstacles, np.array([[i, -10, w, h]])), axis=0)
21 for i in range(-10, 60):
22 obstacles = np.concatenate((obstacles, np.array([[60, i, w, h]])), axis=0)
23 for i in range(-10, 61):
24 obstacles = np.concatenate((obstacles, np.array([[i, 60, w, h]])), axis=0)
25 for i in range(-10, 61):
26 obstacles = np.concatenate((obstacles, np.array([[-10, i, w, h]])), axis=0)
27 for i in range(-10, 40):
28 obstacles = np.concatenate((obstacles, np.array([[20, i, w, h]])), axis=0)
29 for i in range(0, 40):
30 obstacles = np.concatenate((obstacles, np.array([[40, 60 - i, w, h]])), axis=0)
31
32 # create some hazards
33 hazards = np.array([[44, 24, 8, 15, 2], [26, 36, 9, 4, 1.75]])
34
35 # define the bounds of the search region
36 min_pos = np.min(obstacles[:, 0:2], axis=0)
37 max_pos = np.max(obstacles[:, 0:2], axis=0)
38
39 # create the class, and setup the map
40 aStar = AStar()
41 aStar.set_map(min_pos, max_pos, grid_res, obstacles=obstacles, hazards=hazards)
42
43 # turn on beam search and set max number of nodes to remember
44 aStar.use_beam_search = True
45 aStar.beam_search_max_nodes = 30
46
47 # call the algorithm
48 path, cost, fig, frame_list = aStar.plan(
49 start_pos, end_pos, show_animation=True, save_animation=True
50 )
51
52 return frame_list
which gives this as output.
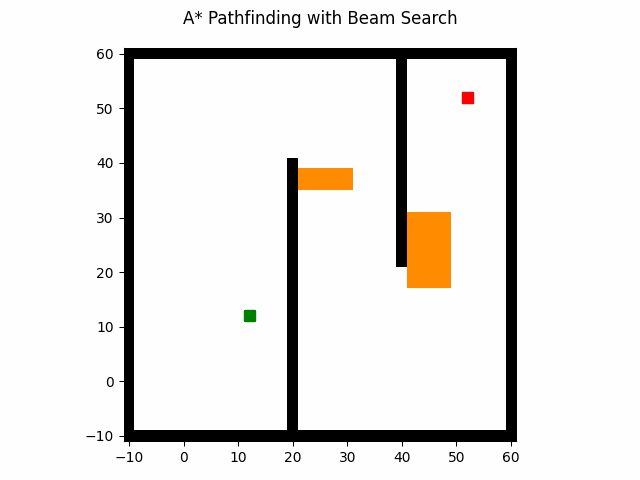
Weighted A*
A modified A* algorithm that weights the heuristic can be run with the following.
1def weighted_a_star():
2 import numpy as np
3 from gncpy.planning.a_star import AStar
4
5 # define start and end location (x, y)
6 start_pos = np.array([10, 10])
7 end_pos = np.array([50, 50])
8
9 # define grid square width/height (x, y)
10 grid_res = np.array([2, 2])
11
12 # create some obstacles
13 obstacles = np.array([])
14 w = 1
15 h = 1
16 for i in range(-10, 60):
17 if obstacles.size == 0:
18 obstacles = np.array([[i, -10, w, h]])
19 continue
20 obstacles = np.concatenate((obstacles, np.array([[i, -10, w, h]])), axis=0)
21 for i in range(-10, 60):
22 obstacles = np.concatenate((obstacles, np.array([[60, i, w, h]])), axis=0)
23 for i in range(-10, 61):
24 obstacles = np.concatenate((obstacles, np.array([[i, 60, w, h]])), axis=0)
25 for i in range(-10, 61):
26 obstacles = np.concatenate((obstacles, np.array([[-10, i, w, h]])), axis=0)
27 for i in range(-10, 40):
28 obstacles = np.concatenate((obstacles, np.array([[20, i, w, h]])), axis=0)
29 for i in range(0, 40):
30 obstacles = np.concatenate((obstacles, np.array([[40, 60 - i, w, h]])), axis=0)
31
32 # create some hazards
33 hazards = np.array([[44, 24, 8, 15, 2], [26, 36, 9, 4, 1.75]])
34
35 # define the bounds of the search region
36 min_pos = np.min(obstacles[:, 0:2], axis=0)
37 max_pos = np.max(obstacles[:, 0:2], axis=0)
38
39 # create the class, and setup the map
40 aStar = AStar()
41 aStar.set_map(min_pos, max_pos, grid_res, obstacles=obstacles, hazards=hazards)
42
43 # set the weight for the heuristic to use, can also override the
44 # aStar.calc_weight function if the weight depends on the node.
45 aStar.weight = 10
46
47 # call the algorithm
48 path, cost, fig, frame_list = aStar.plan(
49 start_pos, end_pos, show_animation=True, save_animation=True
50 )
51
52 return frame_list
which gives this as output.
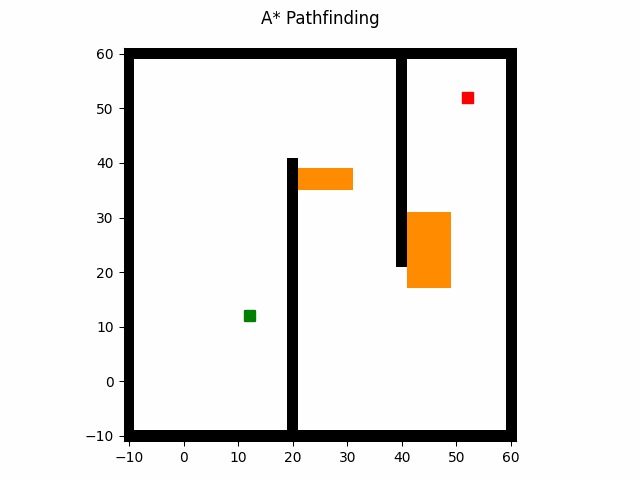